Containerising The Application With Docker
Learn the basics of docker, create simple and multi stage dockerfiles.
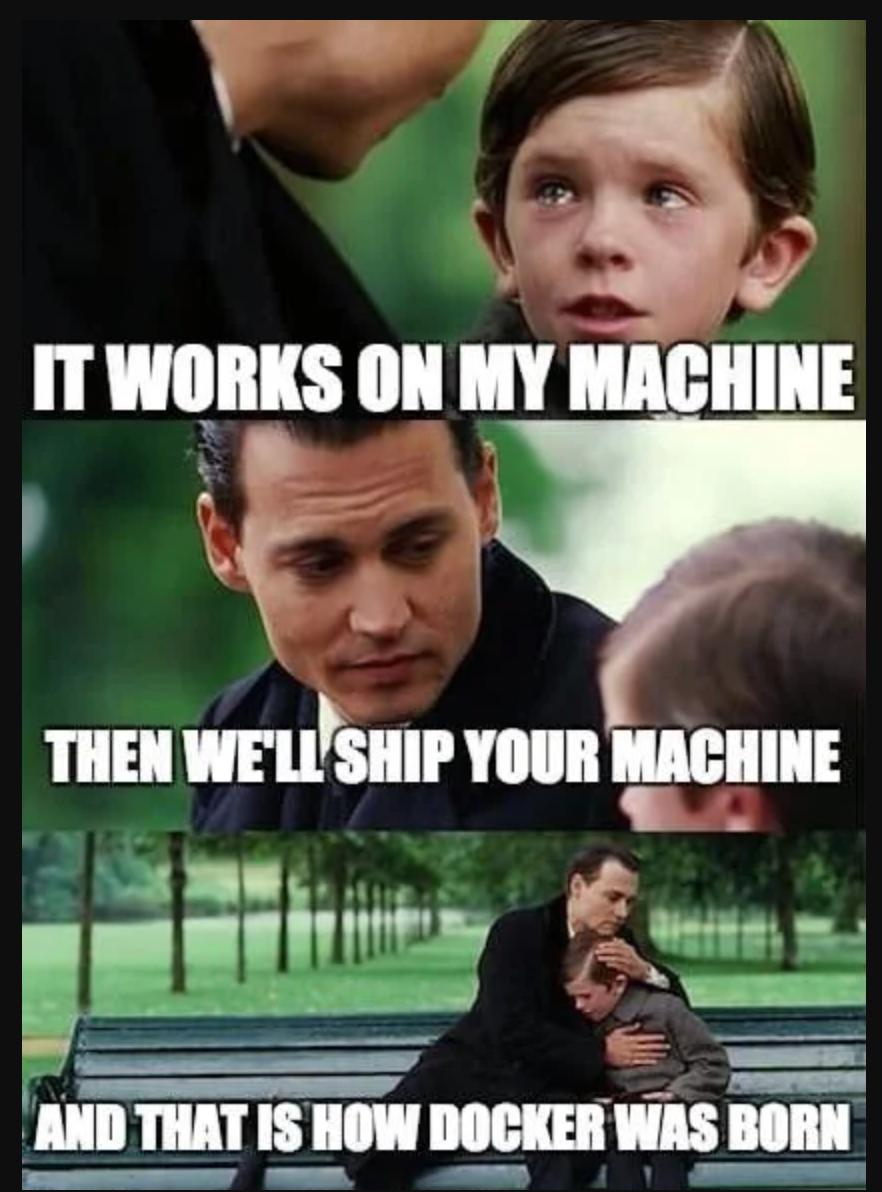
In this article, we are going to learn about Docker and will show you how to containerise the application like a breeze. This will help you to improve application portability, enhance resource utilization and simplify deployment and scaling. Let’s dive in and discover the power of containerization!
What’s Docker and Why Should You Care?
Think of Docker as a magic wand for packaging your applications. It allows you to encapsulate your software, its dependencies, and runtime environment in a neat little container. This means you can run your app anywhere, from your local machine to the cloud, with no surprises.
Step 1: Installing Docker
Before we dive into the fun stuff, you’ll need to install Docker. Head over to the Docker website and grab the installer for your platform.
Step 2: Creating a Dockerfile
A Dockerfile is like a recipe that tells Docker how to build your container. It specifies what operating system, libraries, and code your container needs. Here’s an example for a simple python app:
# Use an official Python runtime as a base image
FROM python:3.8
# Set the working directory in the container
WORKDIR /app
# Copy your local code into the container
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install -r requirements.txt
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Multi-Stage Docker Builds
Ever heard of “building once, running anywhere”? Multi-stage builds in Docker allow you to create smaller, more efficient images. You build your application in one container and then copy only the necessary artifacts into a fresh image.
# Build Stage
FROM node:14 as builder
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production Stage
FROM nginx:alpine
COPY --from=builder /app/build /usr/share/nginx/html
Step 3: Building the Docker Image
Navigate to the directory containing your Dockerfile and run the following command:
docker build -t my-python-app .
This command builds a Docker image named my-python-app based on the instructions in your Dockerfile.
Step 4: Running the Container
Once the image is built, you can run a container based on that image:
docker run -p 4000:80 my-python-app
This command starts a container from the my-python-app image, mapping port 80 in the container to port 4000 on your host machine.
Ansible Configuration for Docker
You can use Ansible to automate the Dockerization process. Here’s an example playbook that automates the steps above:
---
- name: Dockerize Python App
hosts: localhost
tasks:
- name: Copy Dockerfile
copy:
src: Dockerfile
dest: /path/to/your/app
- name: Build Docker Image
command: docker build -t my-python-app /path/to/your/app
- name: Run Docker Container
command: docker run -p 4000:80 my-python-app
And there you have it! With Docker, you can package your applications, dependencies, and environment, making deployment simple.
Add comment
@name